WebSocket Paint
- WunderVision
- Mar 12, 2018
- 3 min read
Updated: Dec 16, 2018
Over the course of the last few days, I have really doubled down on the web projects I have been working on.
The first project I completed using Node.JS is a real time updating paint page that uses websockets to push the draw data to all of the connected clients.
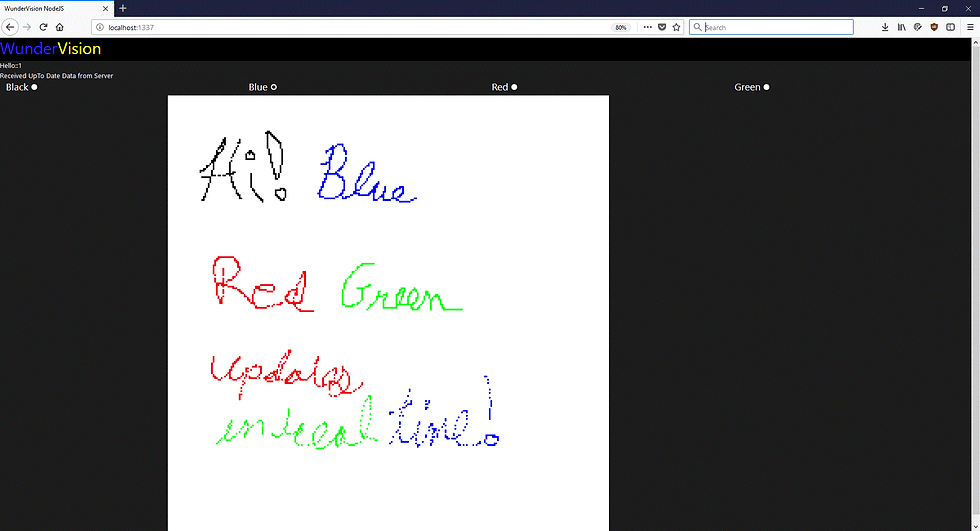
I used Bootstrap to make the page look nice and Socket.IO for the websocket implementation. Websockets allow for 2 way communication between a connected client and the server. This enables the server to push realtime data to all of the clients that are connected, without the client constantly requesting the server for any updates.
Check out the full source code here on github!
Here is a quick demo!
In my last post, I had started off making a simple Node.JS web server. This project just kind of built off of that.
On the server side, I used a couple variables to contain the persistent state.
I used a 2D 1080x1080 array to store all of the color points. The first iteration of this code, I was just sending the raw X,Y coordinate derived from the click/touch on the canvas. This resulted it lots of points being drawn and stored in a list and way to much data passing back and forth. The clients quickly bogged down.
Using a 2D array like this helps quantize the points and still gives the same effect.
One of the main components of this, is to make sure that as a client connects, it gets the most up to date drawing.
I have a callback that handles the refresh call, which is the first thing the client sends to the server. It condenses the 2D array to a list of points and sends it to the client.
I tried just sending the 2D array but it seemed to not be working. Not sure if I was doing something wrong or not.
When the client holds the mouse down or touches the screen, it sends the 'rect' message. The server then determines what the current color is (based on the check boxes), truncates the position to the Size resolution. It then creates a new Shape and places it in the map at the X,Y coordinate. In reality there should be a boundary check there...
One thing I noticed about the Javascript Socket.IO is that broadcast didn't seem to include its own client. Which in some ways makes sense, but that is why it is doing the emit and then the broadcast.emit.
On the client side, it handles the Mouse and Touch events. I added the Touch events using the addEventListener method in script code, and the Mouse events were added in the html.
Here are the callback handlers for the different events. They are pretty straight forward.
One thing you have to take into account is that the offset of the canvas. If you want to get the X,Y coordinates as relative to the canvas itself doing a getBoundingClientRect() gets the coordinates of the canvas. The touches and mouse clicks are based on the page coordinates. So you just subtract the canvas position from the mouse/touch click.
In the Touch callbacks, be sure to call preventDefault(). This makes sure that only the touch calls are handled. It seemed like on Android at least, that the MouseDown would still get called without that call.
All in all this was a fun little project that introduced the web socket ideas and also showed me more of how javascript works. More projects to come
Comments